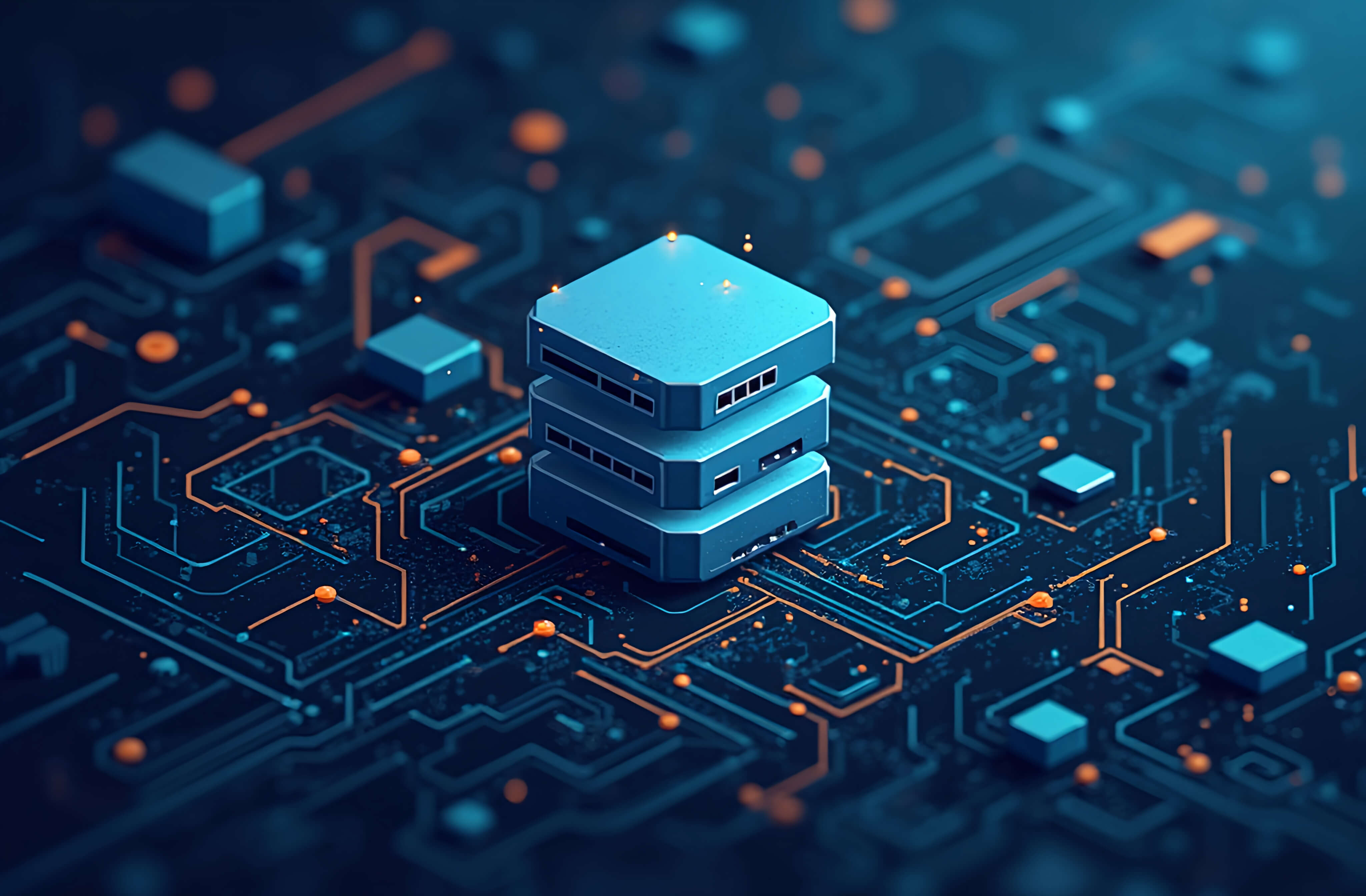
Vector Databases Explained: A Developer's Guide to Modern Similarity Search
In the era of AI-powered applications, traditional databases are no longer sufficient for handling the complex data structures required for semantic search and similarity matching. Vector databases have emerged as a crucial component in modern AI infrastructure, enabling efficient storage and retrieval of high-dimensional data. Whether you're building a recommendation system, implementing semantic search, or developing RAG (Retrieval Augmented Generation) applications, understanding vector databases is essential for any modern developer.
Understanding Vector Embeddings
Before diving into vector databases, it's crucial to understand what they store: vector embeddings. These are numerical representations of data (text, images, audio, etc.) in high-dimensional space, where similar items are positioned closer together. When you convert a piece of text or an image into a vector embedding, you're essentially creating a mathematical representation that captures its semantic meaning.
Vector embeddings transform complex data into a format that machines can understand and compare efficiently, enabling semantic search and similarity matching at scale. For example, the sentences "The cat sat on the mat" and "A kitten rested on the rug" would have similar vector representations despite using different words, because they convey similar meanings. This is fundamentally different from traditional keyword matching and enables more intuitive and powerful search capabilities.
Why Traditional Databases Fall Short
Traditional relational databases excel at exact matches and range queries but struggle with similarity searches in high-dimensional spaces. Consider trying to find similar images in a database:
- Relational DB Approach: Would require exact matches on specific attributes (size, color histograms, etc.)
- Vector DB Approach: Can find similar images based on their overall visual similarity, even if no exact attributes match
The challenge lies in the "curse of dimensionality" - as the number of dimensions increases, traditional indexing methods become inefficient. Vector databases solve this through specialized indexing structures optimized for high-dimensional similarity search.
Popular Vector Database Options
Pinecone: Managed Simplicity
Pinecone has emerged as a popular choice for teams wanting a fully managed vector database solution. Its key advantages include:
- Automatic scaling and optimization
- Simple REST API interface
- Built-in support for common embedding models
- Hybrid search capabilities (combining metadata filtering with vector similarity)
import pinecone
# Initialize Pinecone
pinecone.init(api_key="your-api-key")
# Create an index
pinecone.create_index("product-embeddings", dimension=384)
# Upsert vectors
index.upsert([
("id1", [0.1, 0.2, ...], {"category": "electronics"}),
("id2", [0.3, 0.4, ...], {"category": "clothing"})
])
# Query vectors
results = index.query(
vector=[0.2, 0.3, ...],
filter={"category": "electronics"},
top_k=5
)
Weaviate: Open-Source Flexibility
Weaviate offers a more flexible, open-source approach with unique features:
- GraphQL-based query interface
- Multi-modal data support
- Built-in vectorization modules
- Optional managed cloud service
{
Get {
Product(
near: {
vector: [0.1, 0.2, ...]
certainty: 0.8
}
where: {
operator: Equal
path: ["category"]
valueString: "electronics"
}
) {
name
description
price
}
}
}
ChromaDB: Local Development and Prototyping
ChromaDB has gained popularity for its simplicity and ease of use, especially during development:
- Runs locally with minimal setup
- Python-first API design
- Excellent for prototyping and small to medium datasets
- Easy integration with popular embedding models
import chromadb
# Create a client
client = chromadb.Client()
# Create a collection
collection = client.create_collection("products")
# Add documents
collection.add(
documents=["iPhone 13", "Samsung Galaxy"],
metadatas=[{"category": "electronics"}, {"category": "electronics"}],
ids=["1", "2"]
)
# Query similar items
results = collection.query(
query_texts=["smartphone"],
n_results=2
)
Choosing the Right Vector Database
The choice of vector database depends on several factors:
Scale and Performance Requirements
- Small Scale (< 1M vectors): ChromaDB or local Weaviate
- Medium Scale (1M-10M vectors): Managed Weaviate or Pinecone
- Large Scale (>10M vectors): Pinecone or distributed Weaviate
Deployment Preferences
Feature Requirements
- Multi-Modal Data: Weaviate
- Simple REST API: Pinecone
- GraphQL Support: Weaviate
- Rapid Prototyping: ChromaDB
Applications and Use Cases
RAG Systems
One of the most powerful applications of vector databases is in Retrieval Augmented Generation (RAG) systems. RAG combines the power of large language models with the ability to retrieve and leverage relevant information from vast knowledge sources. Vector databases serve as the backbone for efficient information retrieval in these systems, enabling more accurate and context-aware AI responses.
Semantic Search
Vector databases excel at powering semantic search capabilities, where traditional keyword-based search falls short. By understanding the contextual meaning of queries, these systems can find relevant results even when exact keyword matches don't exist. This is particularly useful in modern AI development where natural language understanding is crucial.
Recommendation Systems
Whether you're building product recommendations, content suggestions, or user similarity matching, vector databases provide the foundation for efficient similarity search at scale. Combined with modern event-driven architectures, these systems can power real-time recommendation engines.
Best Practices for Implementation
Proper Embedding Generation
- Choose appropriate embedding models for your data type
- Maintain consistent embedding dimensions across your application
- Consider using batch processing for large-scale embedding generation
- Implement proper prompting techniques when generating embeddings from language models
Efficient Indexing
- Use appropriate index types for your use case (HNSW, IVF, etc.)
- Balance index build time vs. query performance
- Monitor index size and update frequency
Query Optimization
- Implement proper metadata filtering
- Use appropriate similarity metrics (cosine, euclidean, dot product)
- Optimize batch sizes for bulk operations
Monitoring and Maintenance
- Track query latency and throughput
- Monitor index health and performance
- Implement proper backup and recovery procedures
Future Trends and Considerations
The vector database landscape is rapidly evolving, with several emerging trends:
- Hybrid Search Capabilities: Combining traditional search with vector similarity
- Multi-Modal Indexing: Supporting different types of embeddings in the same index
- Edge Deployment: Running vector search on edge devices
- Improved Compression: More efficient storage of high-dimensional vectors
By understanding these aspects of vector databases, developers can better leverage AI technologies to enhance their applications with advanced search and recommendation capabilities.